We’re choosing a build tool for VS 2008 project.
I’m not expert in any, only had put together a couple of NAnt scripts. So I give no objective technical information in this post, just impressions.
- First discussion of this choice, back in 2005, is almost strictly in favor of NAnt. Though, read it to know about MSBuild differences and advantages.
- Recent blog post by Oren Eini and int comments are also generally for NAnt. Additionally, you can use <msbuild> task in NAnt.
- The latter mentions Yahoo! ALT.NET group discussion. Newsgroup fan can read a lot there, I also had some. There are reasonable votes there for MSBuild because it’s just deployed with .NET, ant it’s nearly functionally equivalent to NAnt.
Though, I can’t forget first discussion that mentioned lack of tasks like editing files or grabbing text output, or incrementing build number – (you know, devil is in details!) – it seems to me that NAnt wins.
Here’s the most useful message there, as for me.
You see, I didn’t go too deep down simple Google search. That was enough for me, you’re welcome to research deeper and to correct me… especially until I started creating build procedure <grin>
And I trust mature and popular opensource products.
Till now, I did understand but didn’t remember the very situation, as I wasn’t the one creating thread pools and alike: all the core things were already there.
It’s a blessing to work for start-up.
You can think about
Monitor.Wait(toRetrieve); // will throw SynchronizationLockException
Now, after a look into docs, I see that one needs to hold the monitored object lock to avoid race conditions: a thread wakes up already holding a lock for an object it needs. The thread that did Pulse()
acquired that lock and safely passed it to Wait()
ing one.
Just watch out for deadlocks: it’s easy to wake up with one object lock, try to acquire another and die then…
lock(toRetrieve)
{
Monitor.Wait(toRetrieve); // will throw SynchronizationLockException
}
As Doug Lea recommends in his excellent eternal “Concurrent Programming in Java“, just make it impossible. Lock only on a single object. If you really really need two locks, make them ordered: always acquire A then B.
If you do:
lock(a){
// :
lock (b)
{
//:
}
// or:
b.SomethingThatLocks();
}
//then will you never ever do
lock(b)
{
//:
a.SomethingThatMightLock();
}
as calling other objects’ methods while holding a lock badly affects your karma.
For more in-detail look, read “EventWaitHandle and Monitors: how to pick one?” by Joe Duffy. He goes very deep down to system details.
He also did a nice brief overview of missed pulse problem – why do you always check wait condition in a loop:
while (toRetrieve.Count == 0)
{
lock(toRetrieve)
{
Monitor.Wait(toRetrieve);
}
}
As it’s common for Java GUI frameworks, only main thread should access GUI objects (see respective MSDN article). Alright, real requirement is a bit weaker: Only the thread that the Dispatcher
was created on may access the DispatcherObject
directly. Effectively same, just keep it in mind.
First thing to know
From other threads, to call main one, do:
someControl.Dispatcher.BeginInvoke(DispatcherPriority.Normal,
invokedMethodDelegate, parameters);
Know that all the BeginInvoke()s go through ThreadPool. .NET’s ThreadPool is static utility class, with a single task queue for everything.
Which means client call can freeze all the application, including WPF internal tasks.
Can you think of any reason to do so? I can’t. It’s another “we’ll never know“.
If you need a thread pool, I recommend a SmartThreadPool by Ami Bar. It can be instantiated, so different pools of tasks won’t clash with each other.
Second thing
Read Kent Boogaart’s post about binding to collections. Modifying or even iterating a collection across threads needs some workarounds, if you can’t afford to lock it entirely. Kent’s .NET blog is worth reading anyway.
One more little thing
is: unhandled exceptions go to <Application DispatcherUnhandledException=”your handler”>. You didn’t expect main thread to handle them, right? Especially as there is no Main()
function… Oh, once I missed that too. So, have a proper handler in each function you start as a thread.
Current “to-reads” are:
Sorry if this looks more like a linkdump; will update when I get more into this. I know I will…
I prefer first to identify program classes, then to think some time about its design, the longer the better.
Then, to prove my internal API idea, I make up code pieces by “wishful thinking”: how I wish the code to look, for it to be the most brief and to express core system objects and functionality, for classes to be least coupled etc etc.
I write them down to code, mock up classes, and try to make the code example work in unit test harness.
During this process I see more details: what does the API miss in order to make pieces work, especially in initialization, finalization, and whatever things were missed during initial design.
Finally I get a (huh) nearly working system core covered with readable unit tests.
I believe, not whe worst approach. Now, how can it be improved?
What did I miss?
- Why FindAll() is not in IList? IDictionary or at least Dictionary? Are you pushing me to code for implementation?
- Why WeakReference, but no WeakDictionary (get a nice one from Nick Guerrera)? WeakList? More?
- Why ReadonlyCollection<T>, but no ReadonlyDictionary<K, V>?
- (I can live with this one) Why Array.Length, but anyCollection.Count (thank Nick Guerrera again)?
…more to follow as I recall them…
Am I the only one who ran into .NET (or GDI?) bug with Pen (or Matrix) .ScaleTransform()?
When I scale a pen to certain width, and it has an anchor, anchor draws at inverse scale.
I might be missing something, but here’s my test. Pen scales proportionally to form width, for simplicity.
Here’s how it looks:
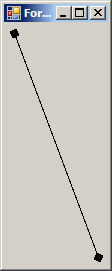
You see, the thinner the line, the bigger is the cap. Uh-oh.
My workaround should be here after some time.
Source code for interested ones:
…
Building a demo for P-Explorer, I got a metaphor, If software project’s:
- architecture – is a skeleton;
- code is meat;
- then it’s blood is work items: new requirements, bugs, change requests.
If you have no skeleton, you’ll most likely to get a slug… or a damn efficient octopus.
Watch out not to get leukaemia – a domination of bugs in blood. Strangely, sometimes it’s a sign of success.
It turned out that a very productive idea — to use domain data scheme (sample) as a basic of requirements categorization — is already used. Moreover, idea is standardized in ISO-13250: Topic Maps.
Wow.
Though, some other, non-domain topics will appear: like nonfunctional requirements, GUI details, project specifics. But still I believe topic map is to be based on domain model.
Got a web reference to an article “Applied MVC Patterns. A pattern language” by Sergey Alpaev.
Model-View-Controller is one of the most complex and pribably the most misinterpreted patterns among GoF’s.
The article is a perfect classification, I recommend it as a must read for everyone who is confused about what is Controller for, and to those who already know it.
Wikipedia article also has some very basic analysis of the pattern.
Till two years ago, I didn’t know that C# 2.0 had closures (reference to Joe Walnes’ blog). Now declarative programming is slowly coming into fashion.
Reference taken from classicist. The latter has a bunch of short bliki-articles, all worth reading.
Please note variables visibility scope:
public List<Employee> HighPaid(List<Employee> emps) {
int threshold = 150;
return emps.FindAll(delegate(Employee e) {
return e.Salary > threshold;
});
}
interesting quotation about arguments against closures in Java – about memory allocation. Found again by Martin Fowler’s reference, in Guy Steele’s archive:
One of the early design principles of Java was that heap allocation occurs if and only if a construct involving the "new" keyword is executed. Adding full-blown closures violated this design principle. (Dynamic class loading also violated the principle, but users seemed to be comfortable with that, perhaps because they believed that "once the computation got going" no more heap allocation would occur.)
Other features for Java release 1.5 will perform certain kinds of autoboxing, which also violates the design principle. This fact will make it easier to argue for restoring full-blown support for closures in the future.
upd: But .NET doesn’t have an unordered container (set), and these cool Find()/FindAll() don’t exist in IList. Too bad.
Oh yes, and Python always had closures.
Discussing Java vs C# coding conventions, I got an idea:
Geek code for a coding conventions.
Like:
-----BEGIN GEEK CODE-CODE BLOCK-----
GP:java,c,cpp,haskell
Off:2S P:N Name:Camel Flex:2
------END GEEK CODE-CODE BLOCK------
which means:
- GP:java,c,cpp,haskell – geek of programming languages (listed);
Off:2S
– prefer 2-space offsets;
P:N
– place parentheses on new line (opposed to S
– same);
Name:Camel
– prefer camelCase;
Flex(0,1,2)
– I’m flexible on this and can easily accept other’s conventions.
This will help others to see what you prefer an not (?) to start stupid holy arguing.